LEDs a Status Indicator
When does the driver of the car know whether he can drive or not? For this we will add 2 leds. As always, green means "you can drive", red means "stop". As long as there is no car in front of the barrier, the red LED is on. Only when the gate is completely open, the green LED is switched on and the red LED is switched off.
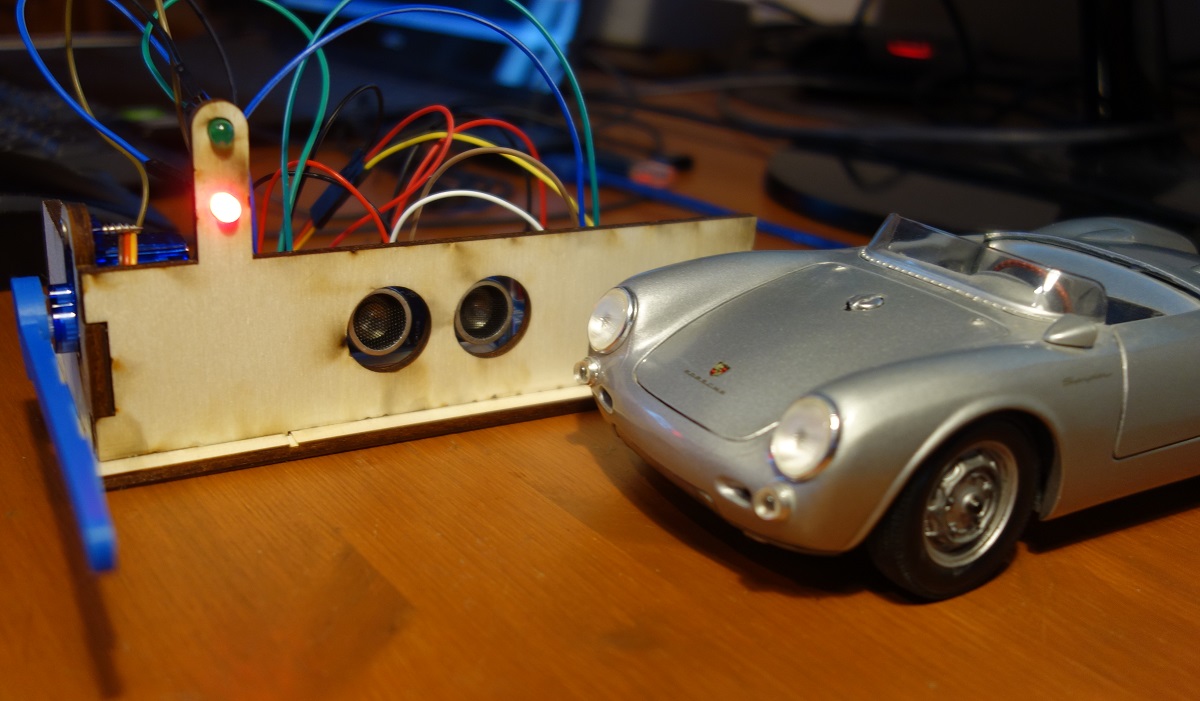
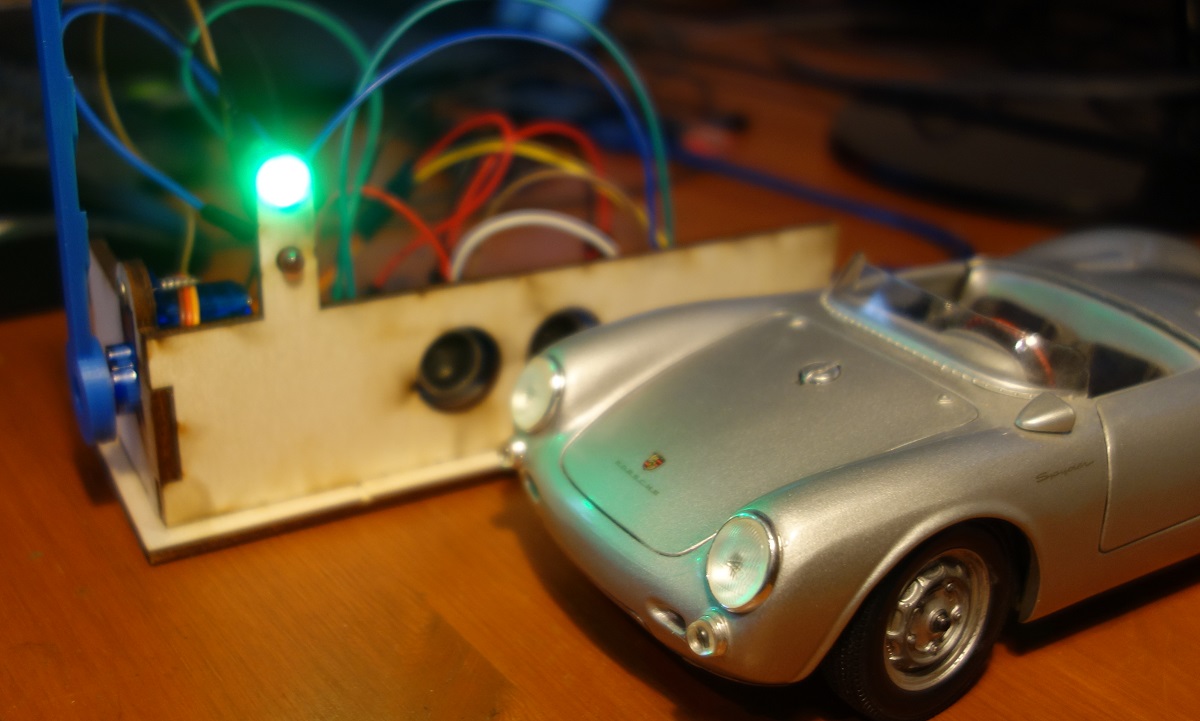
Wiring Diagram
Every LED has a current that it can safely handle. Going beyond that maximum current cound damage the LED. Thus, limiting the current through the LED with the use of a series resistor is a common recommendation. But i have driven many LEDs without resistor with an Arduino and had no problems. But as always: it's your own risk!
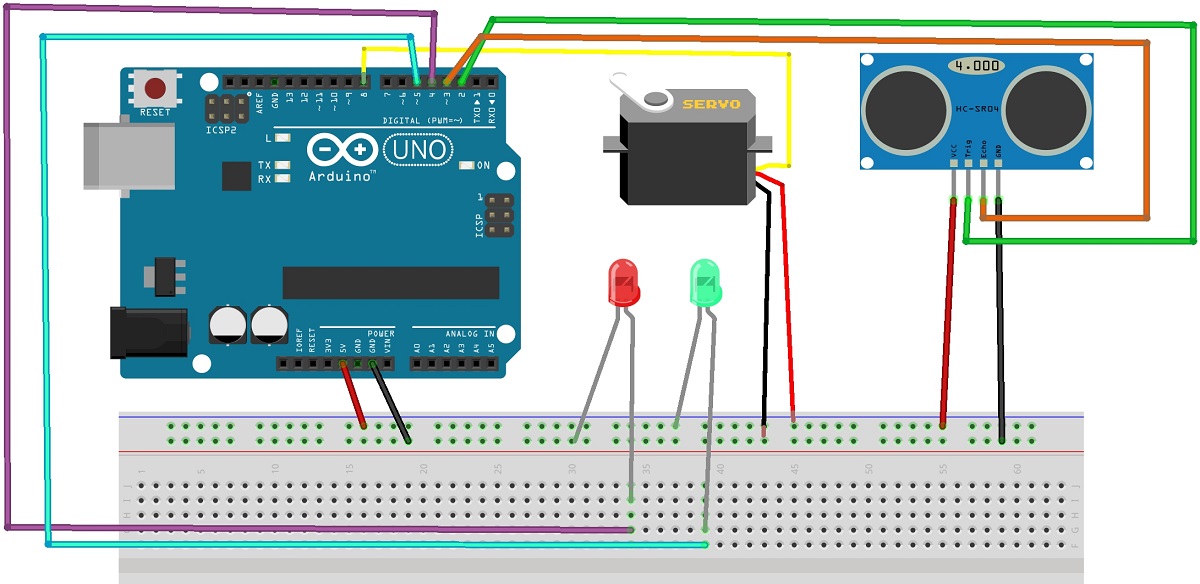
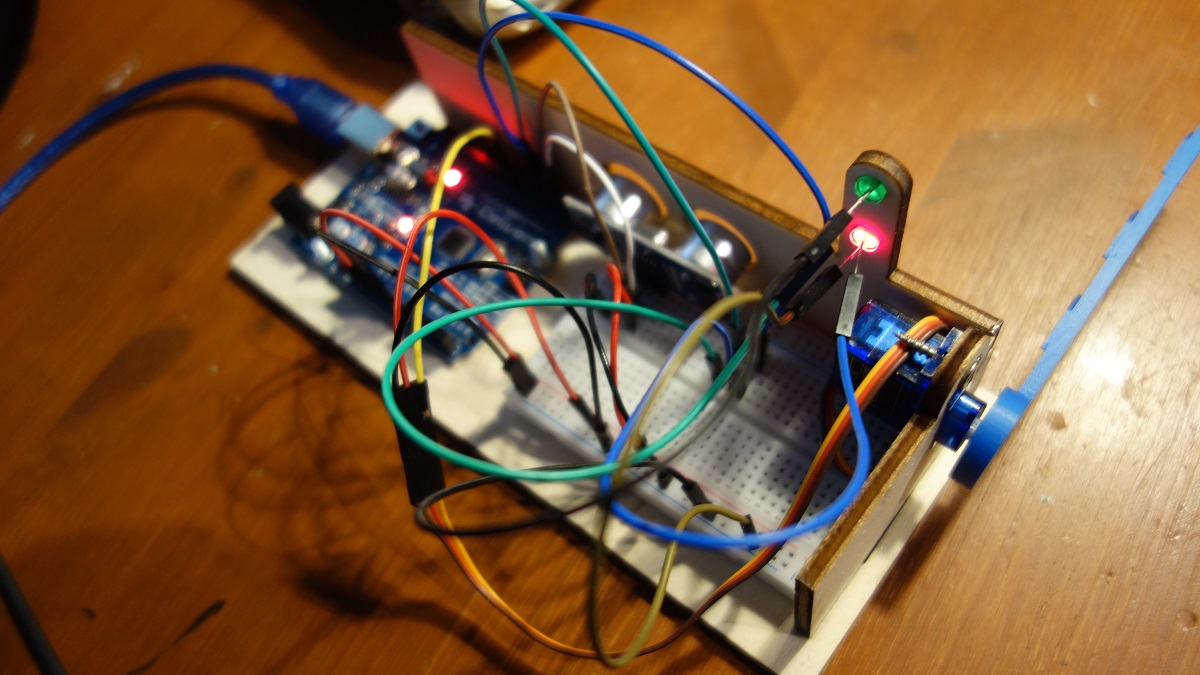
Complete Program for the Smart Gate
/* Smart Gate Tutorial - STEP 3 * smart gate with leds to display the status of the gate * (c) Stefan Hager 2023 */ // You need to include the servo library #include <Servo.h> // create object myservo from class Servo Servo gate; // red LED is on pin 4 int red = 4; // green LED is on pin 5 int green = 5; void setup() { // initialize gate gate.attach(8); // connect Servo with PIN 8 gate.write(90); // use 90 degrees as start value // initialize sensor // set pin 2 = trigger to output, pin 3 = echo to input pinMode(2, OUTPUT); pinMode(3, INPUT); // LEDs pinMode(red, OUTPUT); pinMode(green, OUTPUT); // Leds: red is on, green is off digitalWrite(red, HIGH); digitalWrite(green, LOW); } void loop() { if(carDetected()) { // car detected -> open gate openGate(); digitalWrite(green, HIGH); digitalWrite(red, LOW); while(carDetected()) { // as long es the car is in front of the gate -> wait... delay(200); } // car gone - wait one second and set light to red delay(1000); digitalWrite(green, LOW); digitalWrite(red, HIGH); // wait for 2 more seconds -> then close the gate delay(2000); closeGate(); } // no car detected -> do nothing delay(1000); } bool carDetected() { digitalWrite(2, LOW); delayMicroseconds(2); // Sets the trigPin HIGH (ACTIVE) for 10 microseconds digitalWrite(2, HIGH); delayMicroseconds(10); digitalWrite(2, LOW); // Reads the echoPin (pin 3), returns the sound wave travel time in microseconds int duration = pulseIn(3, HIGH); // Calculating the distance int dist = duration * 0.017; if(dist < 10) return true; return false; } // opens the gate void openGate() { // move slowly from 90 to 180 degrees for(int pos = 90; pos <= 180; pos++) { gate.write(pos); delay(10); } } // closes the gate void closeGate() { // slowly move back for(int pos = 180; pos >= 90; pos--) { gate.write(pos); delay(10); } }