Make it Smarter
A gate that always opens and closes after a few seconds is of course not smart. The next step is therefore to open the gate only when a vehicle really wants to drive through it. To determine whether a vehicle is waiting in front of the gate, we will use an ultrasonic sensor.
The HC-SR04 is a very simple and inexpensive ultrasonic sensor. An ultrasonic sensor sends a signal inaudible to us waits for the echo. From the elapsed time, you can then calculate the distance of the body that reflected the signal.
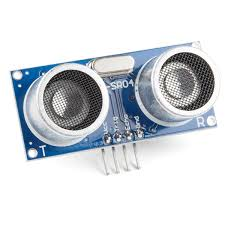
Place the sensor on the breadboard so that the two openings for the ultrasonic transmitter and the receiver fit exactly through the opening.
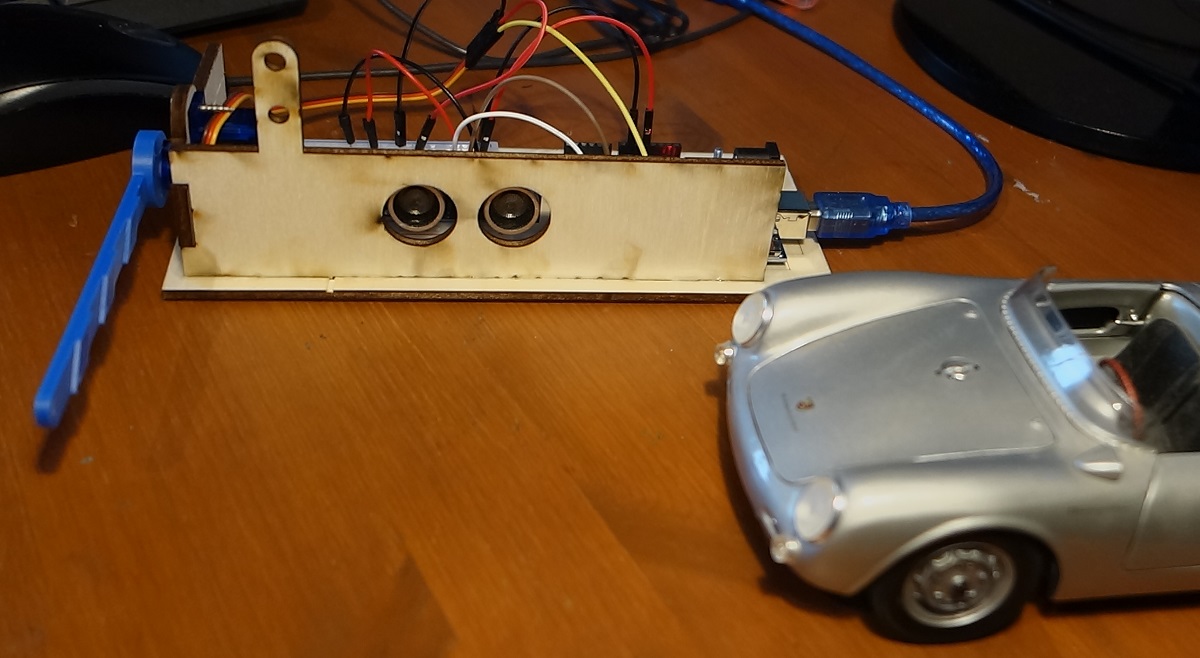
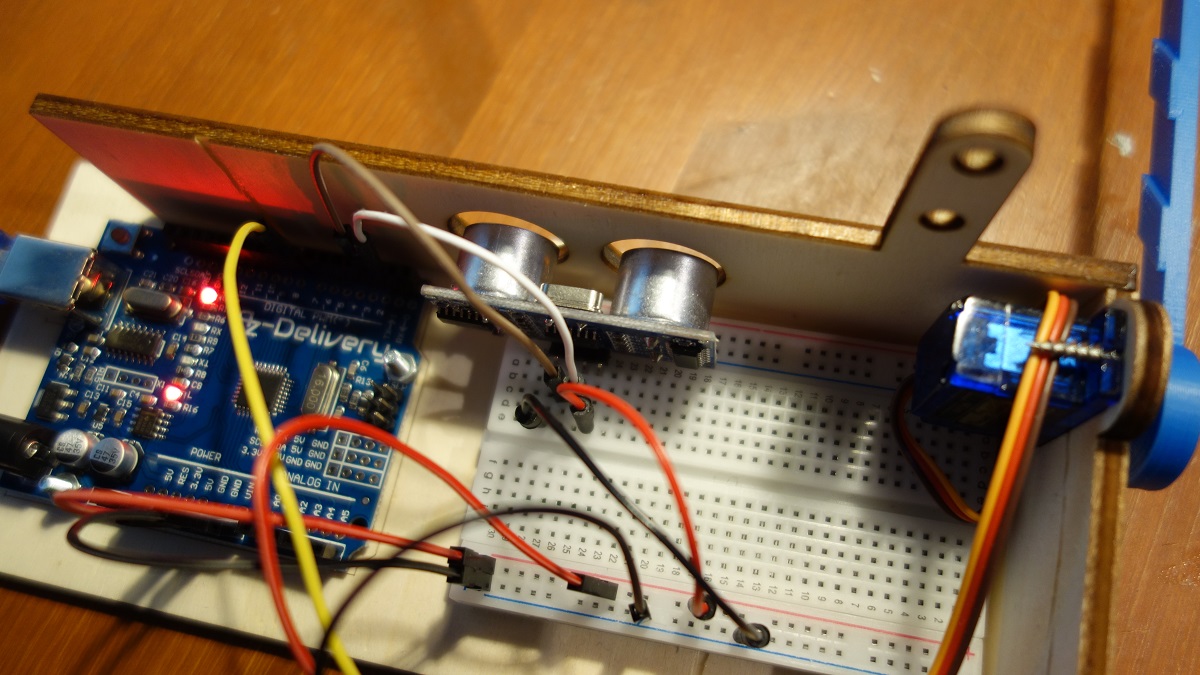
Wiring diagram for HC-SR04
Note: The sensor works with 5V supply voltage and needs very little power. So we can directly use the power supply of the Arduino board. The PIN TRIG on the HC-SR04 is used to trigger the sound signal, the PIN ECHO provides a HIGH signal as soon as the echo is received.
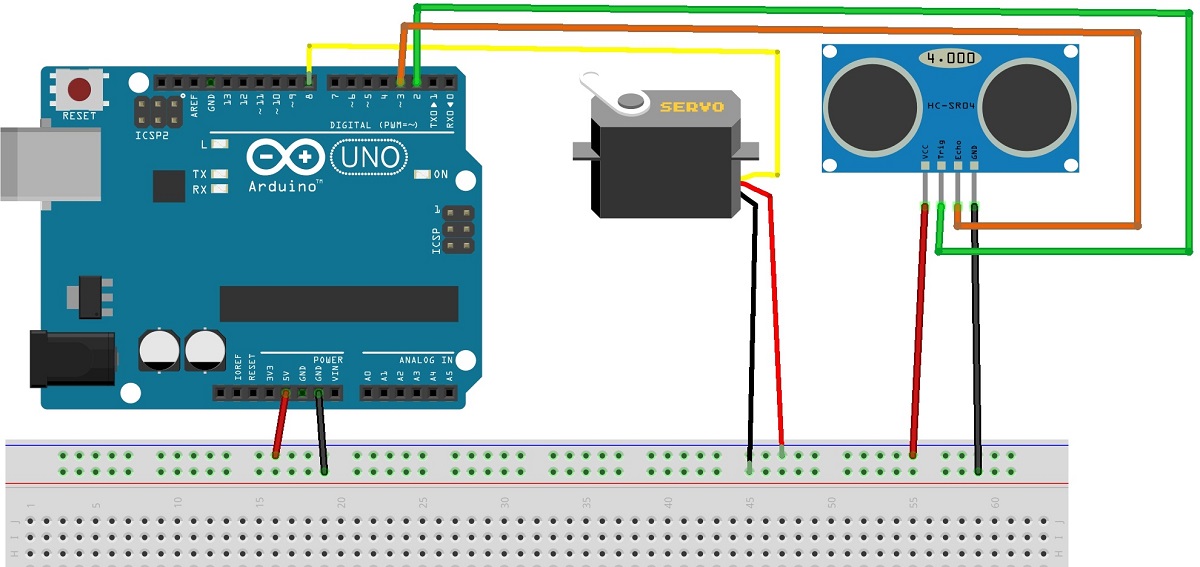
Program for Sensor Test
Use this code to test the sensor, the value for the estimated distance will be displayed in the serial monitor of the Arduino IDE.
/* Smart Gate * Test of ultrasonic sensor * Distance of obstacle will be displayed in the * serial monitor of the Arduino IDE * (c) Stefan Hager 2023 */ void setup() { Serial.begin(9600); Serial.println("Sensor - Test"); // set pin 2 = trigger to output, pin 3 = echo to input pinMode(2, OUTPUT); pinMode(3, INPUT); } void loop() { // Use pin 2 for trigger // Clears the trigPin condition digitalWrite(2, LOW); delayMicroseconds(2); // Sets the trigPin HIGH (ACTIVE) for 10 microseconds digitalWrite(2, HIGH); delayMicroseconds(10); digitalWrite(2, LOW); // Reads the echoPin (pin 3), returns the sound wave travel time in microseconds int duration = pulseIn(3, HIGH); // Calculating the distance int dist = duration * 0.017; // Displays the distance on the Serial Monitor Serial.print("Distance: "); Serial.print(dist); Serial.println(" cm"); // wait half a second delay(500); }
Program for Smart Gate
If the test with the ultrasonic sensor was successful, we can now create the program for the smart gate. For this purpose, the program is extended by a boolean function "carDetected()", which returns "true" if a vehicle was detected, otherwise "false". I assume that a vehicle has been detected if an obstacle in front of the sensor is less than 10 cm away.
/* Smart Gate Tutorial - STEP 2 * Automated gate using a ultrasonic sensor and a servo to open the gate * Opens the gate if car was detected, waits for the pass to pass -> closes * the gate * * (c) Stefan Hager 2023 */ // You need to include the servo library #include <Servo.h> // create object myservo from class Servo Servo gate; void setup() { // initialize gate gate.attach(8); // connect Servo with PIN 8 gate.write(90); // use 90 degrees as start value // initialize sensor // set pin 2 = trigger to output, pin 3 = echo to input pinMode(2, OUTPUT); pinMode(3, INPUT); } void loop() { if(carDetected()) { // car detected -> open gate openGate(); while(carDetected()) { // as long es the car is in front of the gate -> wait... delay(200); } // car gone - wait for 2 more seconds -> close gate delay(2000); closeGate(); } // no car detected -> do nothing delay(1000); } bool carDetected() { digitalWrite(2, LOW); delayMicroseconds(2); // Sets the trigPin HIGH (ACTIVE) for 10 microseconds digitalWrite(2, HIGH); delayMicroseconds(10); digitalWrite(2, LOW); // Reads the echoPin (pin 3), returns the sound wave travel time in microseconds int duration = pulseIn(3, HIGH); // Calculating the distance int dist = duration * 0.017; if(dist < 10) return true; return false; } // opens the gate void openGate() { // move slowly from 90 to 180 degrees for(int pos = 90; pos <= 180; pos++) { gate.write(pos); delay(10); } } // closes the gate void closeGate() { // slowly move back for(int pos = 180; pos >= 90; pos--) { gate.write(pos); delay(10); } }