Matching Commands
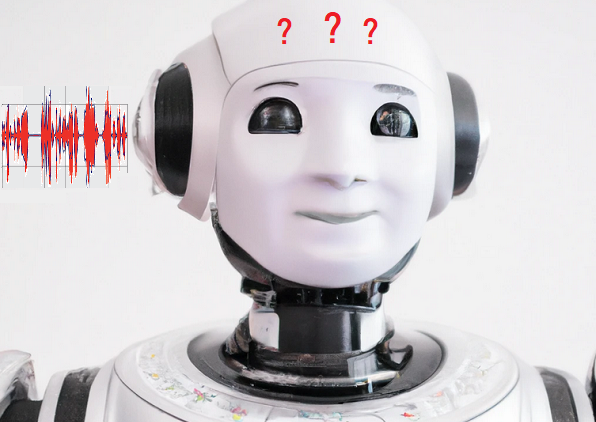
Now it is a matter of recognizing the desired commands in the text generated by deepspeech. If a command is recognized exactly 1:1 by the speech recognition, it is of course easy, but unfortunately words are very often not recognized 100% correct. Example:
>> hallo >>> partial match : hallo r : 0.8
The stored command is "hello", but the string "hallo r" was recognized. To make the detection a little better, I implemented the "CommandMatcher" class.
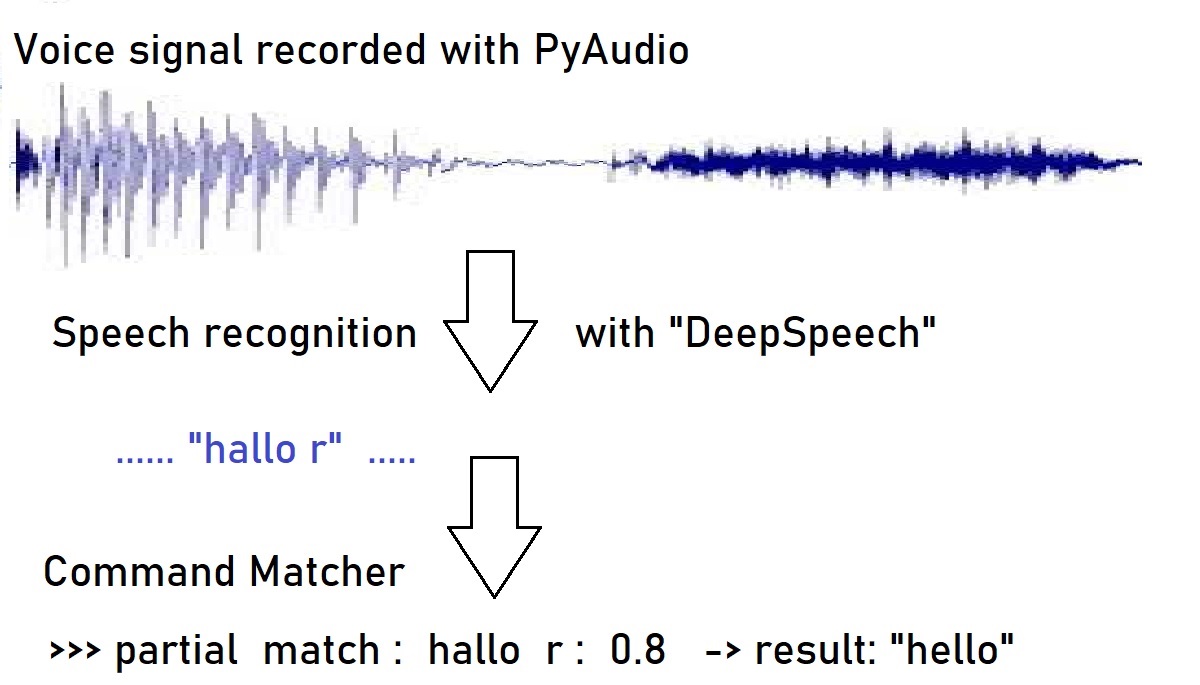
The class has only one static method "matchCommand", which checks the passed text for full and partial matches of commands.
class CommandMatcher: @staticmethod def matchCommand(command, text): if command in text: # direct match print(">>> full match : ",command) return True else: # no direct match comwords = command.split() textwords = text.split() length = len(comwords) if length == 1: # only one command word to match for word in textwords: ratio = SequenceMatcher(None, word, comwords[0]).ratio() if ratio >= 0.75: print(">>> partial match : ", word, " r : ", ratio) return True return False
Currently I have implemented the partial match only for commands that consist of one word. The command recognition could certainly be improved by implementing this strategy for commands with multiple words as well. Then, for example, commands such as "lights on" or "power off" could be recognized more reliably.