Remote Control Receiver
Like the transmitter, the receiver essentially consists of an arduino nano and a NRF24L01 WiFi module. Since the arduino can only handle a voltage of 5 v, but the battery supplies 9 v, the voltage between the battery and the arduino must be reduced from 9 v to 5 V. To do this, we use a LM2596S or any similar DC / DC converter.
Required parts: 1 x Arduino Nano 1 x NRF24 module 1 x 9V battery 1 x LM2596S or similar DC7DC converter
Wiring
NRF24L01 Arduino MOSI D11 MISO D12 SCK D13 CE D9 CSN D8 VCC +3.3 V GND GND
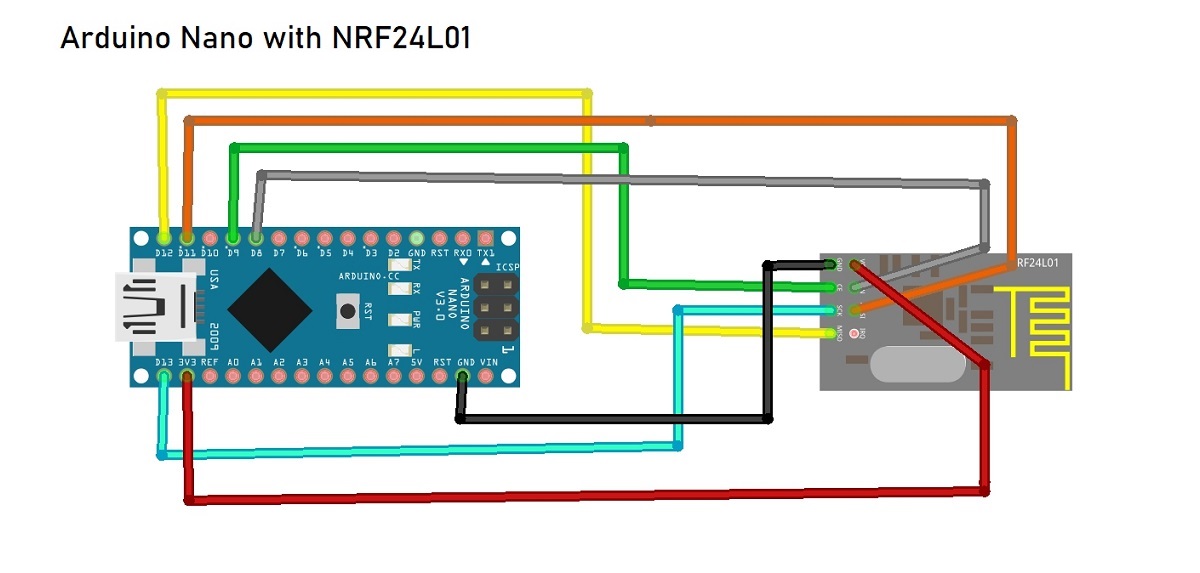
Receiver Software
The software for the receiver allows the boat to move forward and backward and to move the rudder in two steps to the left and right. Driving backwards does not work properly, but theoretically it would be possible.
/* * Airboat RC Controller * Code for transmitter * (c) Stefan Hager 2023 */ #include <SPI.h> #include <nRF24L01.h> #include <RF24.h> #include <Servo.h> #define DEBUG 1 // CE is on pin 9 // CSN is on pin 8 RF24 receiver(9, 8); // servo for airboat rudder Servo rudder; // pins for L298N (motor controller) int PWM = 5; int in1 = 4; int in2 = 3; const byte address[7] = "AIRBOAT"; // this data package is expected from transmitter struct Data_Struct { int thrust = 0; int rudder = 90; }; Data_Struct data; void setup() { Serial.begin(9600); Serial.println("Setup starts...."); // initialize receiver receiver.begin(); if(receiver.isChipConnected()) { Serial.println("Start reading pipe..."); receiver.openReadingPipe(0, address); receiver.setPALevel(0); receiver.setDataRate(RF24_250KBPS); receiver.setChannel(0x60); receiver.startListening(); } else { Serial.println("Chip is not properly connected!"); receiver.stopListening(); } // Servo on pin D5 rudder.attach(6); // move to middle position Serial.println("Positioning rudder servo to 90 degrees..."); rudder.write(90); // initialize L298N pins pinMode(PWM, OUTPUT); pinMode(in1, OUTPUT); pinMode(in2, OUTPUT); } void loop() { // any data...? if(receiver.available()) { receiver.read(&data, sizeof(data)); } // motor if(data.thrust > 60) { // forward digitalWrite(in1, HIGH); digitalWrite(in2, LOW); analogWrite(PWM, data.thrust); Serial.println("F: "); Serial.println(data.thrust); } else if(data.thrust < -60) { // reverse digitalWrite(in1, LOW); digitalWrite(in2, HIGH); analogWrite(PWM, data.thrust); } else { // neutral - switch motor off analogWrite(PWM, 0); } // rudder if(data.rudder < 80 or data.rudder > 120) { // do only move, if you have a value that really differs from neutral (=90) rudder.write(data.rudder); } else { rudder.write(90); } #ifdef DEBUG Serial.print("T: "); Serial.print(data.thrust); Serial.print(" R:"); Serial.println(data.rudder); #endif delay(20); }