STEP 2 : Servo Motors
Using servos is also very simple and works the same way as you would operate the servos directly via the arduino. The only thing you need to know is that the servo connections are connected to pins 9 and 10 of the arduino: Strangely servo_1 is connected to pin 10 and servo_2 to pin 9. All you have to do is plug the servo into servo_1 and upload the sketch.
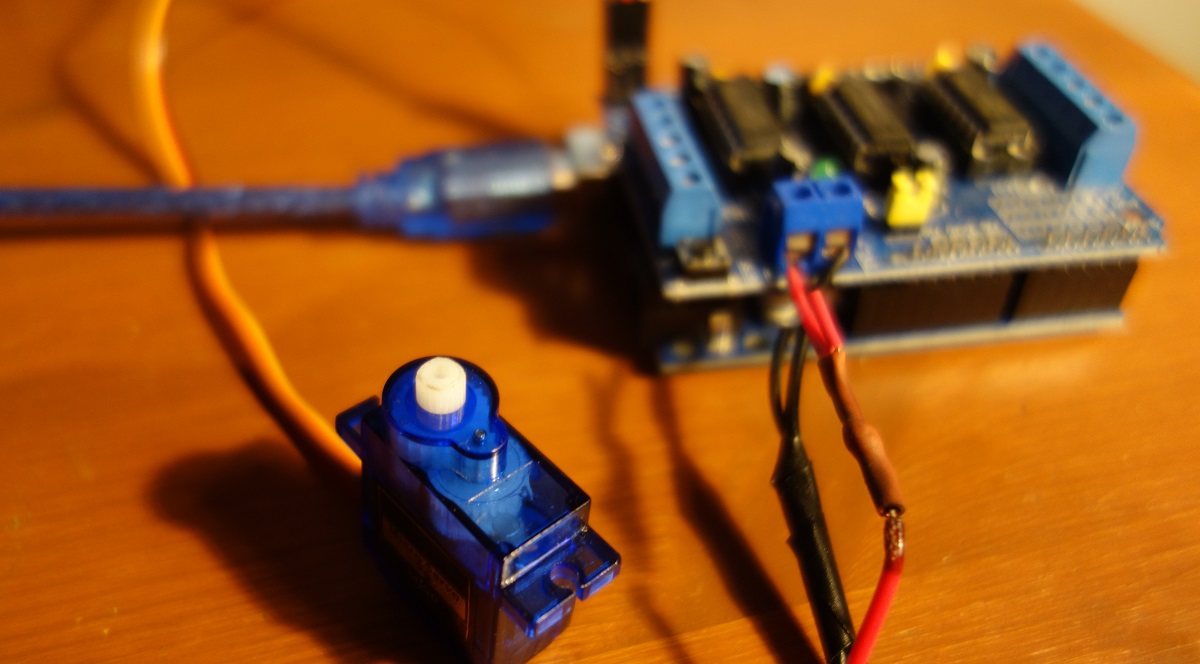
Program to run Servos
The test program is extremely simple: we set the servo to the 90 degree poisition, after 2 seconds we go further to the 180 degrees poisition, then again to 90 degrees, further to 0 degrees and again back to 90 degrees. In each position the servo waits 2 seconds before moving to the next position.
/* Motorshield L293 Tutorial * Running a servo-motor * Stefan Hager 2021 */ // You need to include the servo library #include <Servo.h> // create object myservo from class Servo Servo myServo; void setup() { myServo.attach(10); // connect Servo with PIN 10 (=servo_1) } void loop() { /* * set to 90, 180 and 0 degrees angle, * wait 2 seconds in every new position * step back to 0 again */ myServo.write(90); delay(2000); myServo.write(180); delay(2000); myServo.write(90); delay(2000); myServo.write(0); delay(2000); }