Introduction to the L293 Motorshield
In this tutorial you will learn how to use the motor shield L293 to control DC- and servo- motors with the Arduino. The L293 is a cheap and simple solution for applications which us several DC-motors and servos at the same time. This Arduino shield plugs onto an Arduino and allows to control up to 4 DC motors, 2 stepper motors or 2 servo motors. The H-bridge is the L293D chip which distributes the load. Thus DC motors and power supplies up to 36V can be used.
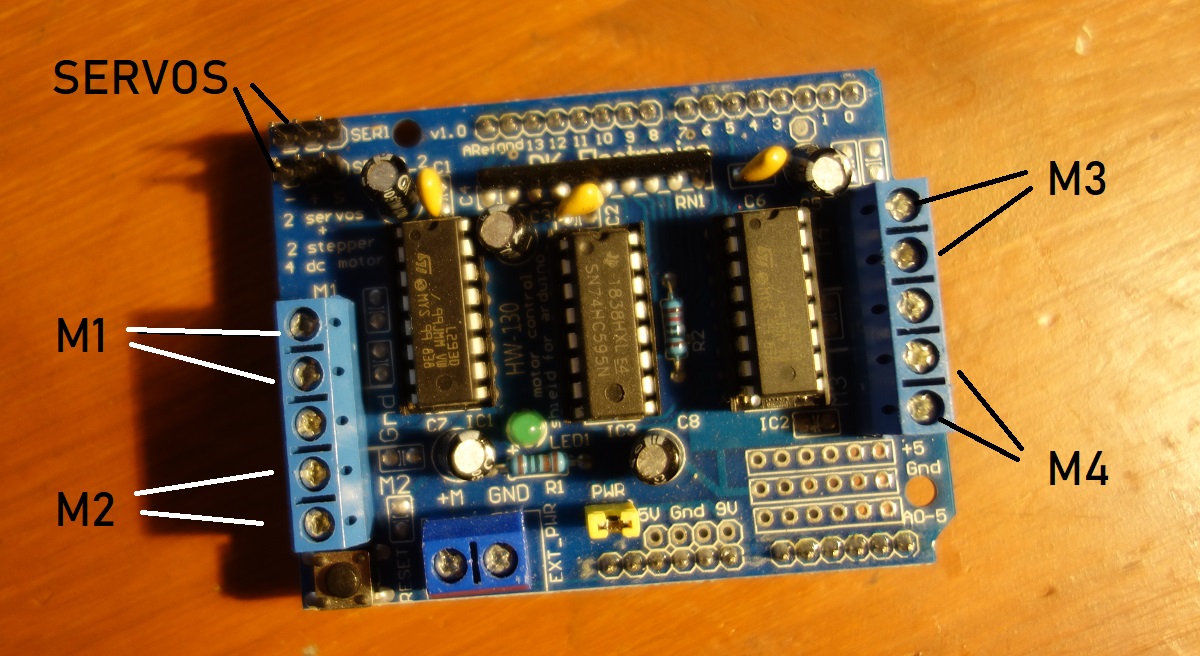
There are 4 connectors for DC motors, marked M1 to M4. Additionally there are connectors servo_1 and servo_2 for 2 servos. To avoid possible damage to the Arduino board on which the shield is mounted, I reccomend using an external power supply that provides a voltage between 7 and 12V.
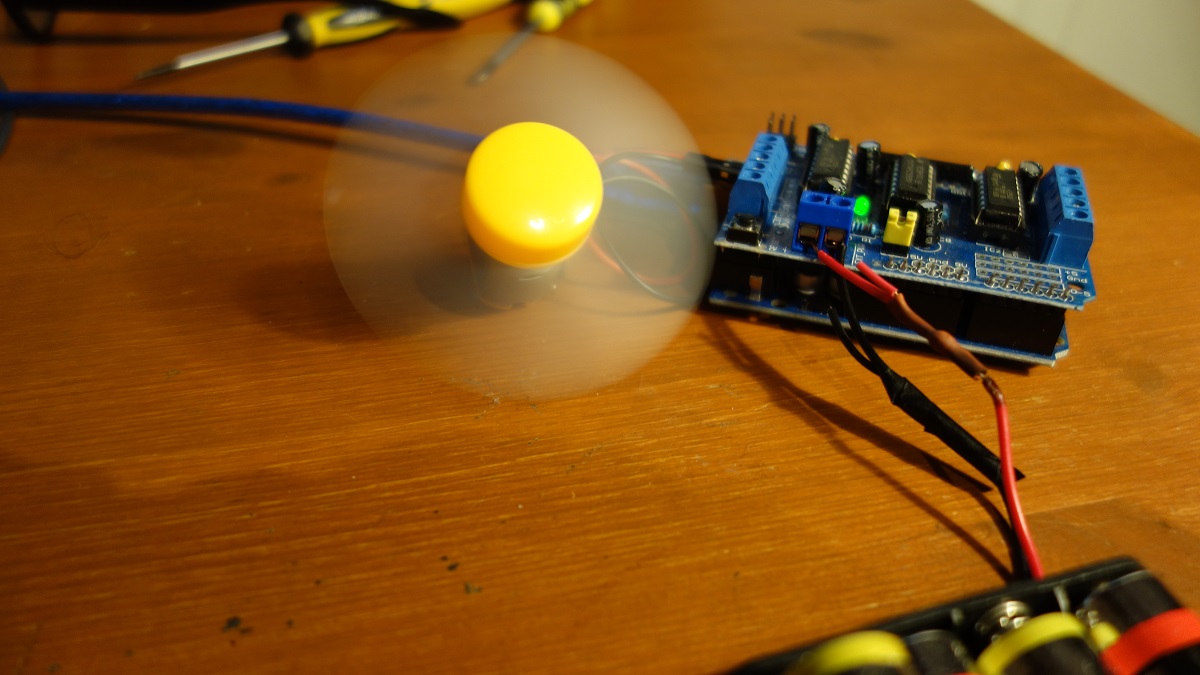
Required Parts
1 x Arduino UNO microcontroller 1 - 2 x Servos (e.g. SG 90) 1 - 4 x DC - motor (4.5 - 36V, max. 1.2A peak) 1 x external power supply (eg. 12V DC supply or 9V battery)
Software Library
There is a special software library for the shield, which makes the control of the dc-motors and the servos extremely easy. Before you can use this library, you have to install it with the library manager of the arduino IDE. You could also directly download the library from e.g. here.
It is even easier to use the library manager: Open the IDE and click to the "sketch" menu and then "include library" > "Manage Libraries". Then the Library Manager will open and you will find a list of libraries that are already installed or ready for installation. If it is not yet installed search for theĀ "adafruit motor shield" library and install it.
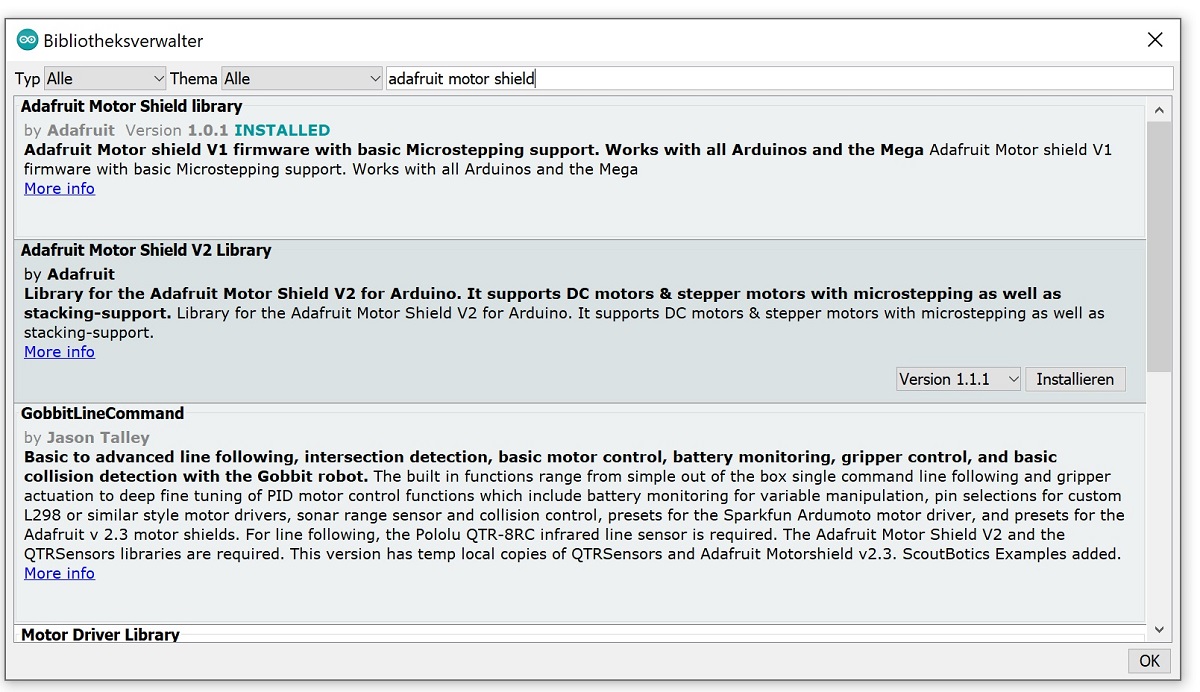
Running DC- Motors
Simply plug the L293 shield into the arduino, then supply is with external power and connect the motor to M1.
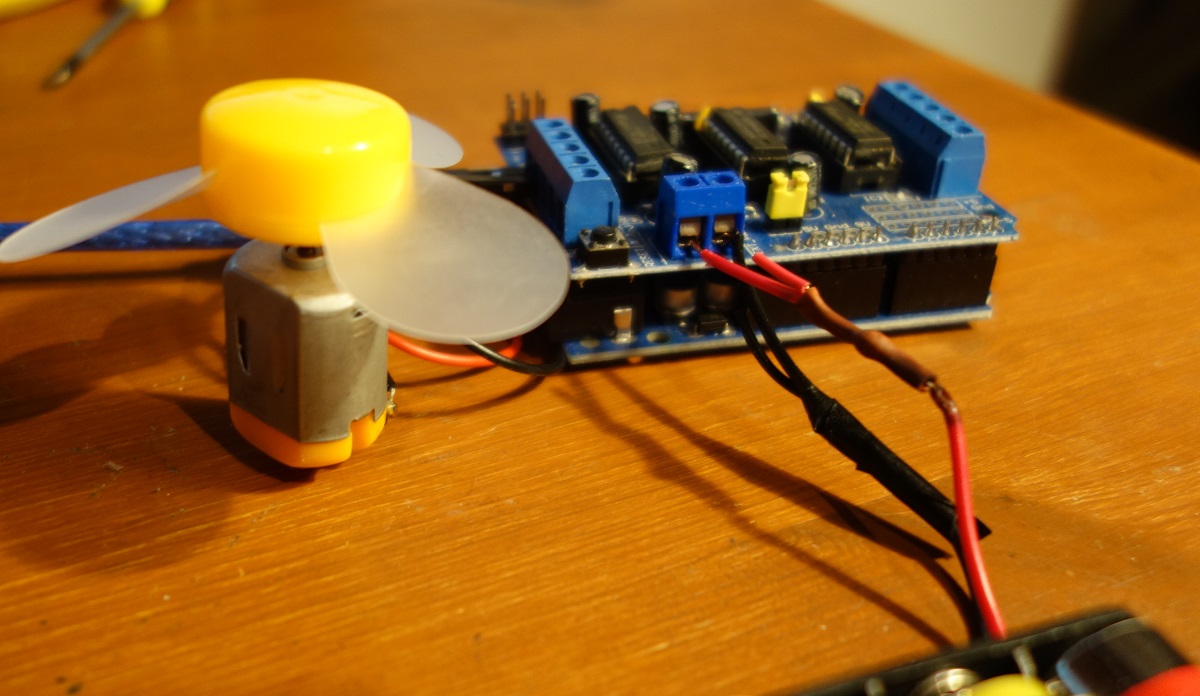
The test program is extremely simple: First an object of type "AF_DCMotor" named "motor" is created. Then the motor runs for 2 seconds forward, then for 2 seconds backward. This is repeated again and again.
/* Motorshield L293 Tutorial * Running a DC-motor * Running forward for 2 seconds, switch direction for 2 seconds and repeat ... * Stefan Hager (c) 2021 */ // this is the include for the adafruit motor shield library #include <AFMotor.h> // we run one motor on M1 (=1) AF_DCMotor motor(1); int speed = 100; // value between 0 and 255 void setup() { Serial.begin(9600); Serial.println("Motor - Test"); motor.setSpeed(speed); } void loop() { motor.run(FORWARD); delay(2000); motor.run(BACKWARD); delay(2000); }