C++ Program (OOP)
If you want to realize more complex projects, it is always advantageous to organize the source code in classes. The C++ class "Sensor" encapsulates all the required functionality for distance measurement. Please note that with C++ you always need 2 files for a class, the header file and the implementation file.
/* Ultrasonic sensor header file * Defines a class for the ultrasonic sensor * (c) Stefan Hager 2022 */ #ifndef _SENSOR_H_ #define _SENSOR_H_ class Sensor { private: int trigg, echo; public: Sensor (int _trigg, int _echo); // constructor int measureDistance(); }; #endif
/* Ultrasonic sensor implementation file * Implements a class for the ultrasonic sensor * (c) Stefan Hager 2022 */ #include "sensor.h" #include "Arduino.h" Sensor::Sensor (int _trigg, int _echo) { trigg = _trigg; echo = _echo; pinMode(trigg, OUTPUT); pinMode(echo, INPUT); } int Sensor::measureDistance () { int dur = 0; // Clears the trigPin condition digitalWrite(trigg, LOW); delayMicroseconds(2); // Sets the trigPin HIGH (ACTIVE) for 10 microseconds digitalWrite(trigg, HIGH); delayMicroseconds(10); digitalWrite(trigg, LOW); // Reads the echoPin, returns the sound wave travel time in microseconds duration = pulseIn(echo, HIGH); // Calculating the distance int dist = duration * 0.017 return dist; }
// Ultrasonic Sensor - Test Program // (c) Stefan Hager 2022 #include "sensor.h" // PIN 2 = trigger PIN 3 = echo Sensor sensor(2,3); void setup() { Serial.begin(9600); Serial.println("Sensor - Test"); } void loop() { int dist = sensor.measureDistance(); // Displays the distance on the Serial Monitor Serial.print("Distance: "); Serial.print(dist); Serial.println(" cm"); delay(500); }
Running the Program
The method "measureDistance" of the class "Sensor" is called once in each loop pass. The determined distance is then displayed in the serial moitor of the Arduino IDE.
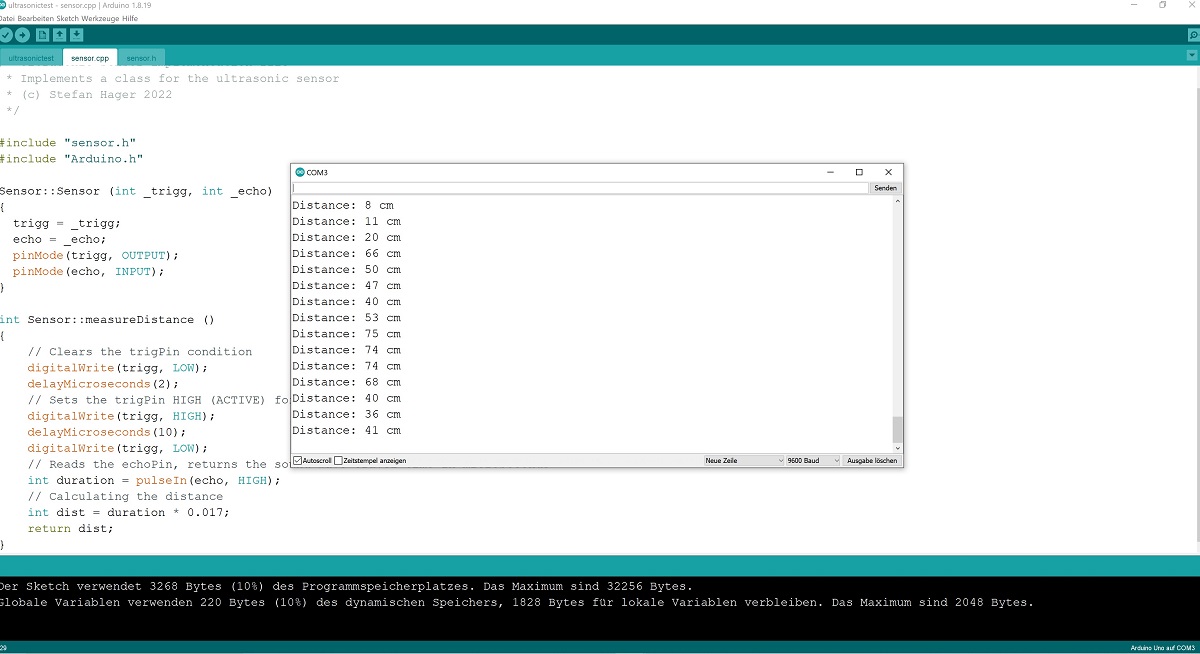