Color-Detection with Arduino
The aim is now to be able to recognize colors from the raw data of the sensor. Please note that the mapping is not generally valid but must be adapted to the respective situation. The pin assignment was taken 1:1 from the functional test.
The main problem with color recognition is to get a suitable mapping from raw data to the colors to be recognized. For a simple mapping please have a look at the source code of the test program (below)
Software for Color Detection
For a test, simply start the program, hold objects with different colors in front of the sensor and read the detected colors on the serial monitor.
/* Color Detection Test with TCR 230 - Sensor * * The function "colorDetection" delivers a numerical value * for the detected color. Currently only white, black, * red, blue and green get mapped but you can add additional * mappings if ou want. * * (c) Stefan Hager 2024 */ //debug switch DISPLAY_VALUES-> defined : display color values #define DISPLAY_VALUES // colors to be detected #define WHITE 1 #define BLACK 2 #define RED 3 #define BLUE 4 #define GREEN 5 #define YELLOW 6 #define UNKNOWN 7 // pinout #define SENSOR_S0 4 #define SENSOR_S1 5 #define SENSOR_S2 6 #define SENSOR_S3 7 #define SENSOR_OUT 8 void setup() { pinMode(SENSOR_S0, OUTPUT); pinMode(SENSOR_S1, OUTPUT); pinMode(SENSOR_S2, OUTPUT); pinMode(SENSOR_S3, OUTPUT); pinMode(SENSOR_OUT, INPUT); // Setting frequency-scaling to 20% digitalWrite(SENSOR_S0, HIGH); digitalWrite(SENSOR_S1, LOW); Serial.begin(9600); } void loop() { int color = colorDetection(); if(color == WHITE) Serial.println("White"); if(color == BLACK) Serial.println("Black"); if(color == RED) Serial.println("Red"); if(color == GREEN) Serial.println("Green"); if(color == YELLOW) Serial.println("Yellow"); if(color == BLUE) Serial.println("Blue"); if(color == UNKNOWN) Serial.println("no color detected"); delay(1000); } int colorDetection() { digitalWrite(SENSOR_S2, LOW); digitalWrite(SENSOR_S3, LOW); int red = pulseIn(SENSOR_OUT, LOW); // Reading the output frequency // Setting GREEN filtered photodiodes to be read digitalWrite(SENSOR_S2, HIGH); digitalWrite(SENSOR_S3, HIGH); int green = pulseIn(SENSOR_OUT, LOW); // Setting BLUE filtered photodiodes to be read digitalWrite(SENSOR_S2, LOW); digitalWrite(SENSOR_S3, HIGH); int blue = pulseIn(SENSOR_OUT, LOW); #ifdef DISPLAY_VALUES // Printing RED value on the serial monitor Serial.print("R="); Serial.print(red); Serial.print(" "); // Printing GREEN value on the serial monitor Serial.print("G="); Serial.print(green); Serial.print(" "); // Printing BLUE value on the serial monitor Serial.print("B="); Serial.print(blue); Serial.println(" "); #endif // mapping the colors, i have defined two threshold for more flexility int threshold = 65; int threshold2 = 45; // white delivers ver low values for each color int whitelimit = 40; if(red < threshold && green > threshold && blue > threshold) return RED; if(red > threshold && green > threshold && blue < threshold) return BLUE; if(red > threshold && green < threshold && blue > threshold) return GREEN; if(red > threshold && green > threshold && blue > threshold) return BLACK; if(red < whitelimit && green < whitelimit && blue < whitelimit) return WHITE; // no color detected return UNKNOWN; }
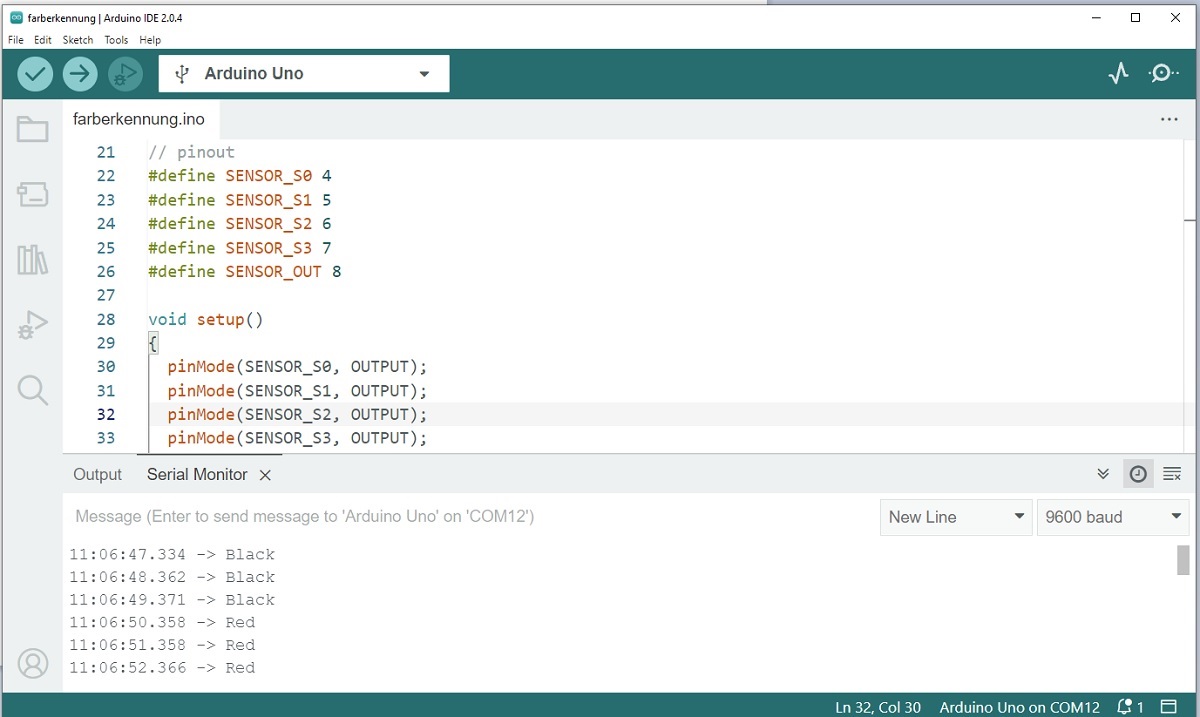