Arduino WIFI Communication
As soon as the transmitter and receiver are ready, the next step is the programming of the software.
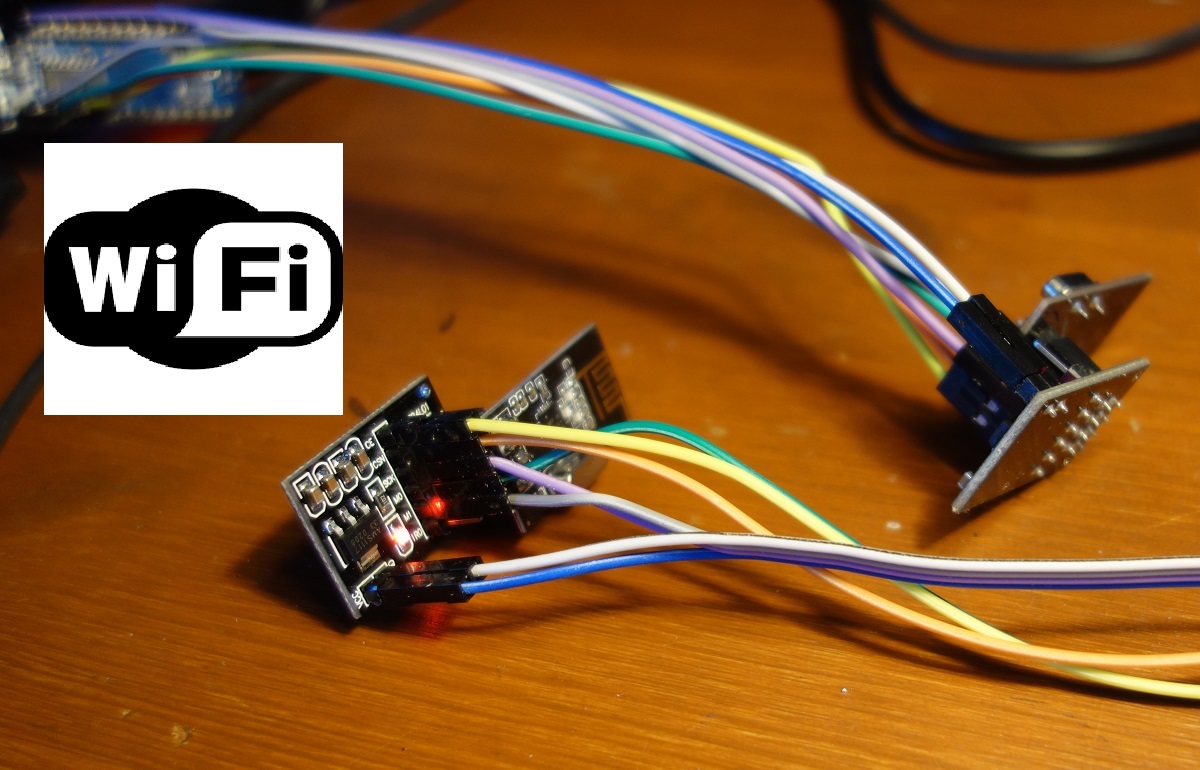
Software Setup
First we need to download and install the RF24 library which makes the programming much easier. We can install this library directly from the Arduino IDE Library Manager. Just search for “rf24” and install “TMRh20, Avamander”.
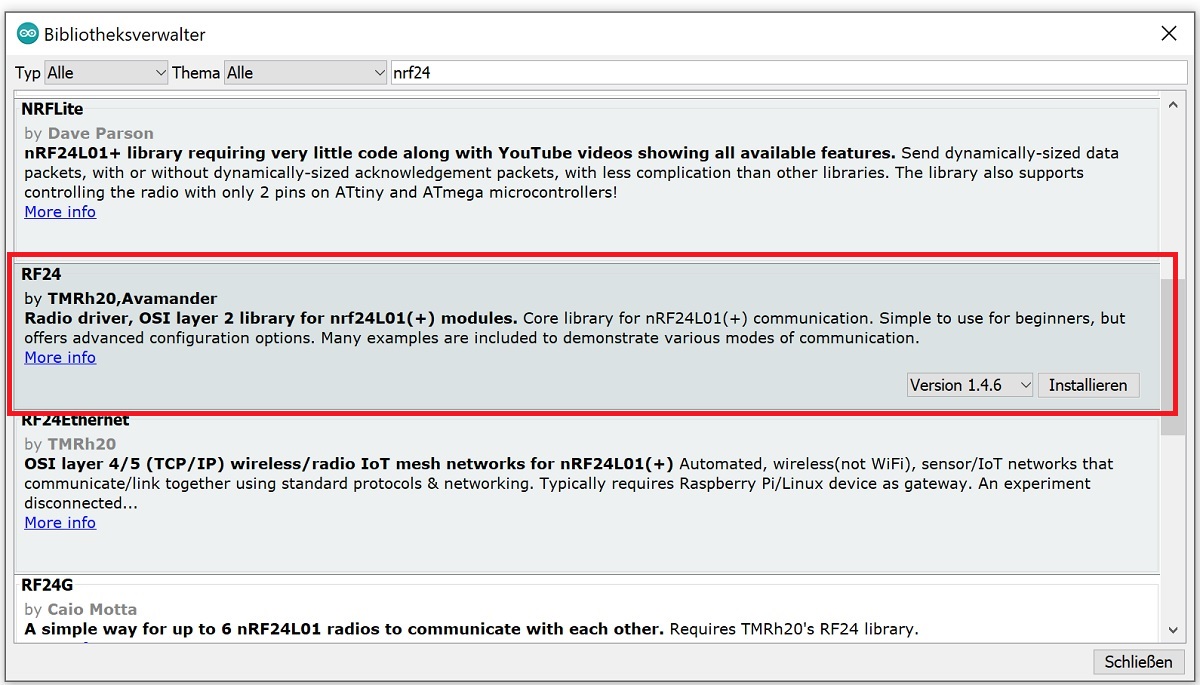
/* * Arduino WIFI Tutorial * Code for Sender * (c) Stefan Hager 2023 */ #include <SPI.h> #include <nRF24L01.h> #include <RF24.h> // CE is on pin 9 // CSN is on pin 8 RF24 sender(9, 8, 2000000); const byte address[6] = "123456"; void setup() { Serial.begin(9600); sender.begin(); if(sender.isChipConnected()) { Serial.println("Sender starting..."); sender.setChannel(0x60); sender.openWritingPipe(address); // important if you power the NRF24 via arduino, higher power levels // consume too much power sender.setPALevel(0); sender.setDataRate(RF24_250KBPS); sender.stopListening(); } else { Serial.println("No chip found...."); } } void loop() { const char text[] = "Hello here is your message..."; sender.write(&text, sizeof(text)); Serial.println("Sending a message..."); delay(1000); }
/* * Arduino WIFI Tutorial * Code for receiver * (c) Stefan Hager 2023 */ #include <SPI.h> #include <nRF24L01.h> #include <RF24.h> // CE is on pin 9 // CSN is on pin 8 RF24 receiver(9, 8, 2000000); const byte address[6] = "123456"; void setup() { Serial.begin(9600); Serial.println("Setup starts...."); receiver.begin(); if(receiver.isChipConnected()) { Serial.println("Start reading pipe..."); receiver.openReadingPipe(0, address); receiver.setPALevel(0); receiver.setDataRate(RF24_250KBPS); receiver.setChannel(0x60); receiver.startListening(); } else { Serial.println("Chip is not properly connected!"); receiver.stopListening(); } } void loop() { if(receiver.available()) { char text[32] = ""; receiver.read(&text, sizeof(text)); Serial.print("Message: "); Serial.println(text); } }
Running the Program
Start the sender first. The start the receiver program and open the serial monitor. You should then see a new message every second.
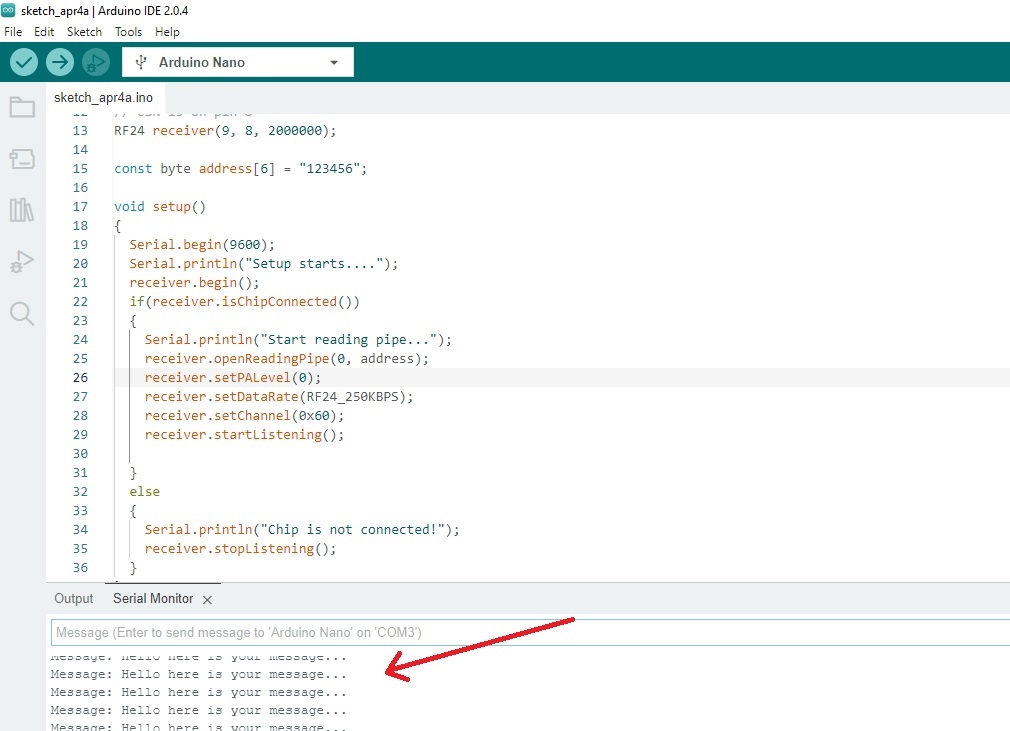