IR-Sensor with Arduino
An infrared module is often used in robots and other automated systems to scan the environment to avoid touching obstacles or to follow lines or other markers. In this tutorial we use the infrared sensor to recognize a black line on white ground, as is often used in robots that are supposed to follow a line.
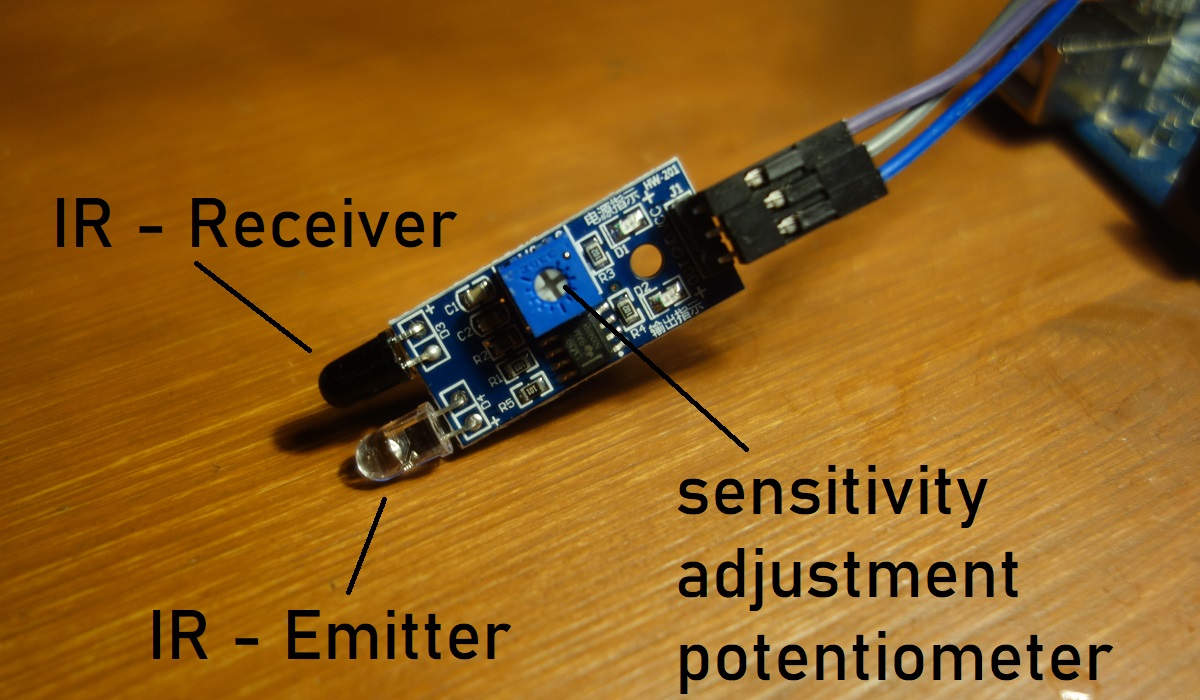
Infrared sensors consist of two elements, a transmitter and a receiver. The transmitter is basically an IR LED, which produces the signal and the IR receiver is a photodiode, which senses the signal produced by the transmitter. The IR sensors emits the infrared light on an object, the light hitting the black part gets absorbed thus giving a low output but the light hitting the white part reflects back to the transmitter which is then detected by the infrared receiver.
Required parts: 1 x Arduino Uno (or compatible) 1 x IR Sensor 3 x jumper cable male - female
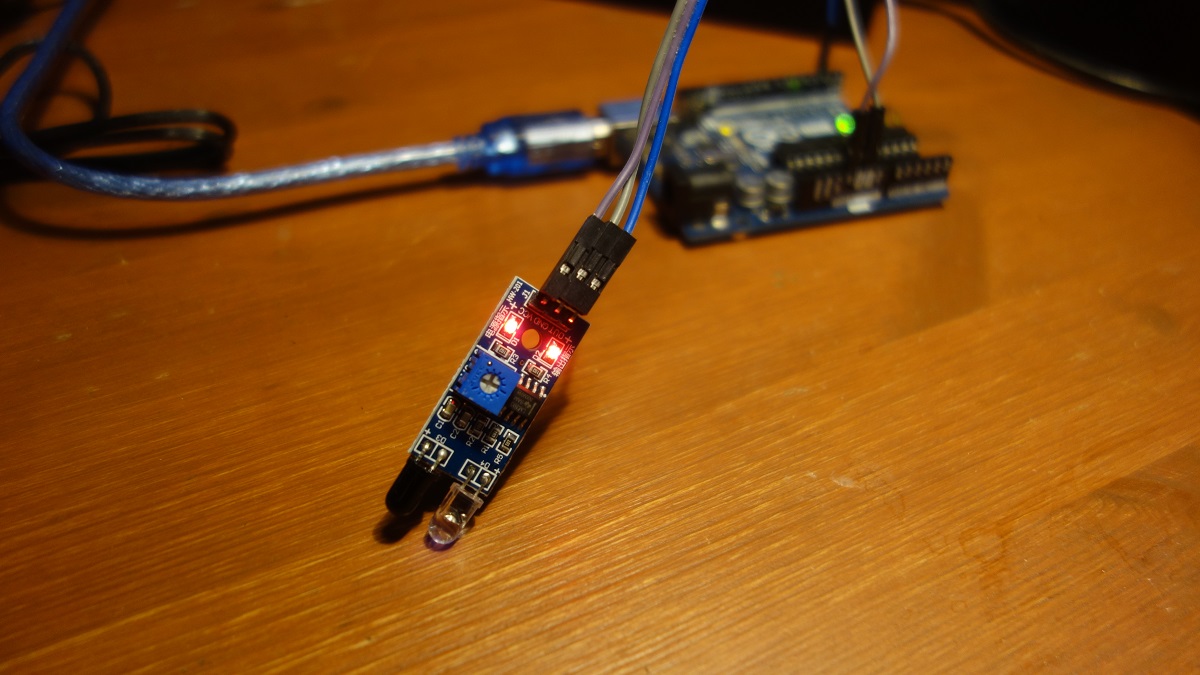
How it works
The infrared sensor is one of the simplest sensors for detecting obstacles and is based on the physical principle of the reflection of light. A source emits light and a receiver detects the intensity of the reflected light. The proportion of reflected light depends not only on the distance between the sensor and the surface, but also on the surface texture, color and orientation of the surface. This is why simple IR sensors are only suitable for obstacle detection or line detection and only to a very limited extent for distance determination.
Wiring Diagram for IR - Sensor
Arduino IR-Sensor ----------------------- 5V VCC GND GND 2 OUT
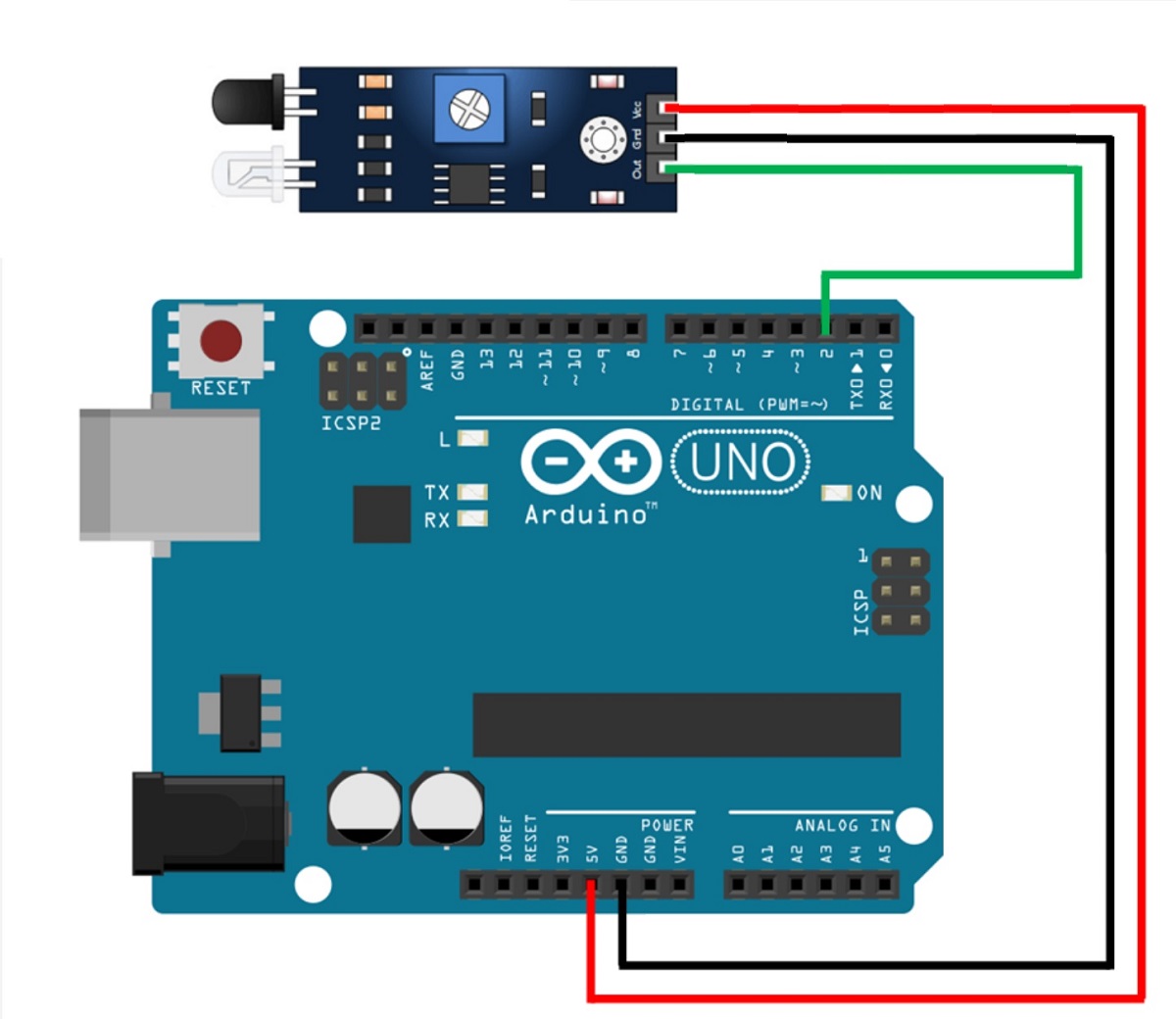
Software for IR-Sensor Test
/* Line Detection with IR - Sensor * prints the word "line" to serial monnitor if a black line is detected * no print output in case the sensor is not positioned over a line * * (c) Stefan Hager 2024 */ int irsensorpin = 2; // This is our input pin for the IR Sensor void setup() { pinMode(irsensorpin, INPUT); Serial.begin(9600); } void loop() { int linedetected = digitalRead(irsensorpin); if (linedetected == HIGH) { Serial.println("line... "); } delay(400); }
Running the Program
Start the program and open the serial monitor. Move the sensor back and forth between the white area and the black line. If the sensor is above the dark line, the output "line..." must appear on the monitor, if you are above the light area, no output appears. Use the potentiometer to set the sensitivity appropriately.
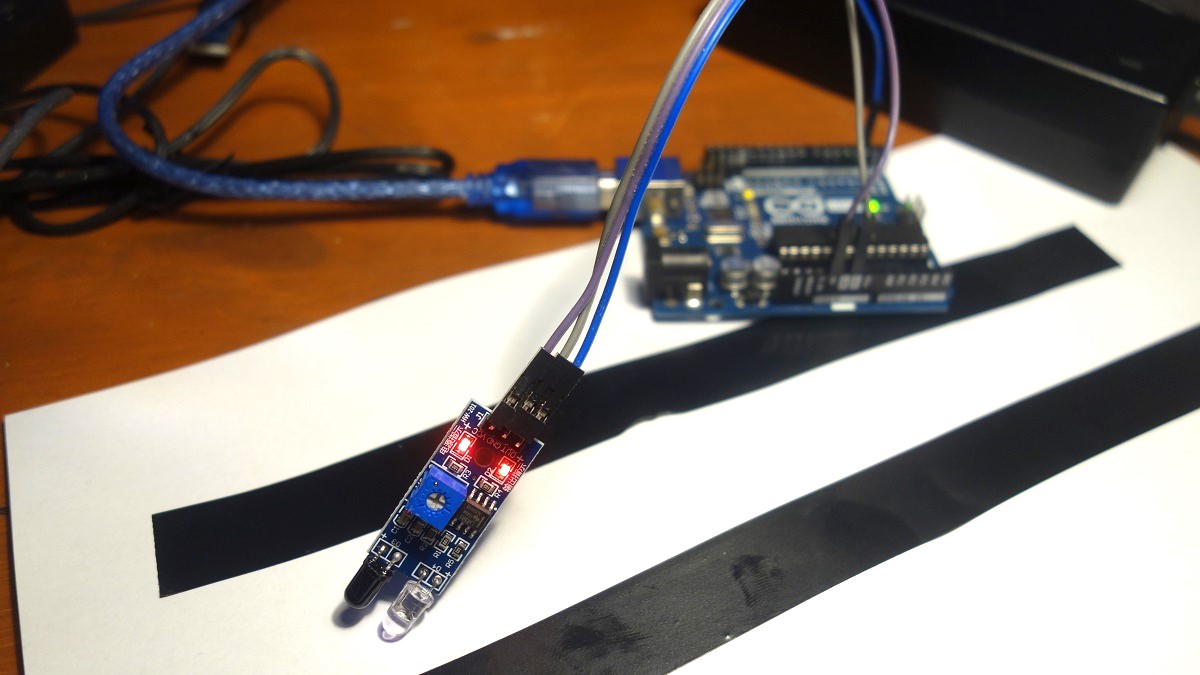